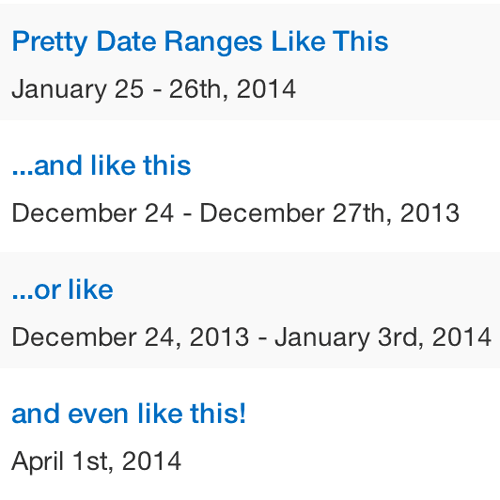
Pretty PHP Date Ranges
Posted on December 28, 2013
Last week I needed to come up with a way to display a pretty date range based on a starting and ending date in PHP. It’s simple enough use PHP’s date function to convert a unix timestamp into a pretty date format, but PHP doesn’t have a built in function to format date ranges.
The goal was to take two unix time stamps and output a range that looks like this:
January 25 – 26th, 2014
This sounds and is simple until you take into account that sometimes it crosses months and needs to look like this:
January 25 – February 2nd, 2014
or could cross years like this:
December 25th, 2013 – January 3rd, 2014
So here is the code to build those prettified date ranges:
* @since 1.0
*/
function jb_verbose_date_range($start_date = '',$end_date = '') {
$date_range = '';
// If only one date, or dates are the same set to FULL verbose date
if ( empty($start_date) || empty($end_date) || ( date('FjY',$start_date) == date('FjY',$end_date) ) ) { // FjY == accounts for same day, different time
$start_date_pretty = date( 'F jS, Y', $start_date );
$end_date_pretty = date( 'F jS, Y', $end_date );
} else {
// Setup basic dates
$start_date_pretty = date( 'F j', $start_date );
$end_date_pretty = date( 'jS, Y', $end_date );
// If years differ add suffix and year to start_date
if ( date('Y',$start_date) != date('Y',$end_date) ) {
$start_date_pretty .= date( 'S, Y', $start_date );
}
// If months differ add suffix and year to end_date
if ( date('F',$start_date) != date('F',$end_date) ) {
$end_date_pretty = date( 'F ', $end_date) . $end_date_pretty;
}
}
// build date_range return string
if( ! empty( $start_date ) ) {
$date_range .= $start_date_pretty;
}
// check if there is an end date and append if not identical
if( ! empty( $end_date ) ) {
if( $end_date_pretty != $start_date_pretty ) {
$date_range .= ' - ' . $end_date_pretty;
}
}
return $date_range;
}
Also available on GitHub/Gist here.
To actually use that in WordPress you’d just add something like this in your template file where you wanted to output the date, here based on two custom field values.
';
echo jb_verbose_date_range( $start_date , $end_date );
echo '';
}