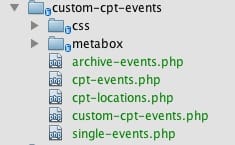
Including templates inside a plugin
Posted on December 17, 2012
This past week I was working on a project for a client who needed a custom post type to manage events on his site. Normally for a project like this I would build the CPTs and push a couple templates in to the active theme folder. But in this instance, the client was working on a new theme that would be going live in a few weeks. Rather than making the client jump through hoops and move template files around, I wanted to provide the easiest solution possible. That meant, including all the templates as part of the plugin. Thanks to a very handy filter in WordPress, this wasn’t a problem. Here’s how it ended up.
Here’s the makeup of the plugin:
The plugin itself is very standard. One thing I like to do is define the custom post types as separate files to keep the function file clean. I then include the CPTs and the custom metabox library like so:
/** Add custom CPTs and Metaboxes */
require_once( 'cpt-events.php' );
require_once( 'cpt-locations.php' );
require_once( 'metabox/init.php' );
I store the stylesheet in a separate folder for cleanliness as well and encode it like this:
/** Enqueue CSS */
add_action( 'wp_enqueue_scripts', 'prefix_add_my_stylesheet' );
function prefix_add_my_stylesheet() {
wp_register_style( 'cpt-style', plugins_url( 'css/style.css', __FILE__) );
wp_enqueue_style( 'cpt-style' );
}
And now the real meat of it. You can see the single-events.php and archive-events.php that make up the two template files I want to use on the front end. What I’m going to do is create a function that tells WordPress to use my templates for the archive and single pages for the event CPT. That code looks like this:
// force use of templates from plugin folder
function cpte_force_template( $template )
{
if( is_archive( 'events' ) ) {
$template = WP_PLUGIN_DIR .'/'. plugin_basename( dirname(__FILE__) ) .'/archive-events.php';
}
if( is_singular( 'events' ) ) {
$template = WP_PLUGIN_DIR .'/'. plugin_basename( dirname(__FILE__) ) .'/single-events.php';
}
return $template;
}
add_filter( 'template_include', 'cpte_force_template' );
As you can see, I’ve set up a filter for template_include. I pass in the $template variable and check to see if the page is either an archive or singular event. If so, I override the default template location with the full path and file name of the file inside my plugin folder.
The end result is a fully encapsulated plugin that takes care of the functionality, templates and stylesheet formatting the client requested all in one easy to manage package. Simply install the plugin and activate it and you’re ready to go!