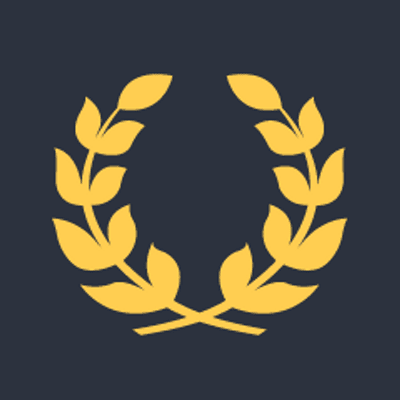
The tools you use, the way you want. Wrapping Help Scout in Nativefier
Posted on July 15, 2016
One of the things we love about WordPress is that it lets us affordably build custom functionality into business’ websites that not long ago would have required tens if not hundreds of thousands of dollars invested in custom software development costs. Which is to say we think people should be able to have software their way.
Wrapping Slack “Making WordPress” in Nativefier
In that vein I was delighted when Jason Cosper wrote last week about using Nativefier to create a mini app for the Making WordPress Core Slack Team.
Like so many businesses these days we use and have quickly come to rely heavily on Slack for our internal team communications. Increasingly however we’re also using it for external communications to keep in touch with specific groups, largely in the tech space but outside it as well. I’ve heard of families starting their own Slack Team just to keep in touch.
Personally I belong to 14 Slack Teams: 5 WordPress groups, 7 non-WordPress technology and local community groups, 2! groups dedicated to just to coffee and a few others. It’s crazy.
One of those WordPress groups is “Making WordPress”, which is a massively huge group of over 10,000 members. It’s a vital communication channel though for everyone involved in the broader WordPress Community. Everything from Core development to organizing WordCamp events to updates to the WordPress.org website to securirty alerts gets discussed there.
Less to it’s core mission it’s both a great way to keep in touch with friends made at WordCamps as well as a great back channel between agencies working in the WordPress space.
Jason Cosper discovered the cause of something that had been driving me crazy the last few months with the native Slack app though. It had become extremely unstable It was frequently crashing and taking up 100% cpu. That’s really frustrating when it’s something you rely on nearly every minute of your work day.
It turns out a big cause of that was including “Making WordPress” and it’s 10,000+ members in the native app. Jason removed that channel and wrapped it up in it’s own desktop web app using a new tool called Nativefier. It’s much like Fluid and what we called an SSB (Site Specific Browser) apps if you ever used those back in the day. Since removing Making WordPress from my native Slack app, like Jason, I’ve found it to be much snappier and hasn’t crashed once.
The now isolated Nativefier app version of Making WordPress still gives me critical desktop notifications of direct messages, plus has the added benefit of staying out of my way most of the day. As you might imagine with 10,000+ members it can be a bit noisey. It’s a win win.
Wrapping Helpscout Making WordPress in Nativefier
It occurred to me I could do the same thing with a few websites I like to keep open all the time. Starting with Help Scout which we use for tracking and responding to plugin support requests.
Steps for Mac OS X
Step 1:
Install Nativefier.
Ok, it’s a bit more complicated. You need to have Node NPM installed first, but if you’re a developer of any kind you probably already do have that. Then just at the command line:
npm install nativefier -g
Step 2:
Wrap up a web page in Nativefier, I did this in ~/Applications and then moved it to /Applications.
nativefier --name "Help Scout" "https://secure.helpscout.net"
Step 3 (optional):
It’ll grab the favicon by default, but his is often low res and ugly. Replace it with a nicer icon.
I used https://iconverticons.com/online/ to convert a Help Scout PNG I scrped from their site into the ICNS format I needed for the dekstop app. You can grab them here for Help Scout if you want: https://iconverticons.com/icons/93a786b5f69feea3/ or create your own.
Final Step:
Right click on the new app and select Open. You’re going to need to right click open on it the first time because it’s not an officially signed by Apple app, but you created it so you can probably decide trust it when the pop-up warning about it being untrusted comes up.
Now if the < 5 minutes it takes to go through those steps yourself is too much, or you don’t have NPM installed, or if your command line shy. You can download my Nativefier wrapped up app of the Help Scout website here: Nativefier – Help Scout.
I can imagine others using this for Gmail, Calendars, all sort of things… Let us know what you’ve used it for in the comments!